In today's rapidly evolving tech landscape, where cross-language compatibility is crucial, mastering this powerful combination can set your applications apart from the competition. The ability to seamlessly transition between Swift's modern features and Objective-C's established ecosystem has become increasingly valuable as many organizations maintain legacy codebases while embracing new development paradigms. As the iOS development community continues to grow, the demand for efficient interoperability between Swift and Objective-C has never been higher. Swift enums, with their powerful pattern matching capabilities and type safety, offer a sophisticated approach to managing application states and complex data relationships. When combined with Objective-C's proven architecture, developers can create more robust and maintainable applications. This guide will walk you through the essential aspects of implementing Swift enums in Objective-C projects, from basic integration to advanced use cases, ensuring you have the tools and knowledge to elevate your development practices. Whether you're working on a new project or maintaining existing code, understanding "swift enum objc" implementation can significantly improve your development workflow and application quality. The importance of mastering Swift enums in Objective-C environments extends beyond just technical implementation. As the iOS ecosystem continues to evolve, developers who can effectively bridge these two languages gain a competitive edge in the market. This comprehensive guide will explore various strategies and best practices for integrating Swift enums into Objective-C projects, providing you with practical solutions to common challenges and innovative approaches to application architecture. From basic syntax considerations to advanced design patterns, we'll cover everything you need to know to become proficient in "swift enum objc" development, ensuring your applications are both efficient and future-proof.
Table of Contents
- Understanding Swift Enum in Objective-C: What Makes It Unique?
- How Does Swift Enum in Objective-C Work?
- What Are the Benefits of Using Swift Enum in Objective-C?
- What Are the Common Challenges with Swift Enum in Objective-C?
- Best Practices for Implementing Swift Enum in Objective-C
- Advanced Techniques in Swift Enum Objective-C Integration
- How Are Swift Enums Used in Real-World Objective-C Projects?
- What Does the Future Hold for Swift Enum in Objective-C?
Understanding Swift Enum in Objective-C: What Makes It Unique?
Swift enums, often referenced as "swift enum objc" in cross-language contexts, represent a fundamental shift in how developers approach data modeling and state management in iOS applications. Unlike traditional enumerations found in other programming languages, Swift's implementation offers a powerful combination of type safety and flexibility that sets it apart in the Objective-C ecosystem. At its core, a Swift enum allows developers to define a common type for a group of related values while providing additional capabilities such as associated values and methods. This enhanced functionality enables developers to create more expressive and maintainable code structures, particularly when interacting with Objective-C components. The technical architecture of Swift enums in Objective-C environments reveals several key features that contribute to their growing popularity. First and foremost, Swift enums can be seamlessly bridged to Objective-C through the @objc attribute, allowing them to be used in legacy codebases while maintaining their modern capabilities. This interoperability is achieved through the generation of NS_ENUM or NS_OPTIONS structures in Objective-C, preserving the type safety benefits while ensuring compatibility with existing code. The ability to define raw values, which can be automatically converted to and from Objective-C types, provides developers with flexibility in how they integrate these enums into their projects. Additionally, Swift enums can include computed properties and methods, enabling developers to encapsulate related functionality directly within the enum itself. When comparing Swift enums to their Objective-C counterparts, several distinct advantages become apparent. Traditional Objective-C enums are essentially integer constants, offering limited functionality beyond simple value representation. In contrast, Swift enums can incorporate associated values, allowing developers to attach additional information to each case. This feature proves particularly valuable when modeling complex states or error conditions in applications. Furthermore, Swift enums support protocol conformance, enabling them to participate in protocol-oriented programming patterns that enhance code reuse and modularity. The ability to define recursive enums and utilize pattern matching adds another layer of sophistication to Swift's implementation, making it possible to create more elegant and concise solutions to common programming challenges in Objective-C projects. The practical implications of these differences become especially relevant when considering modern application development requirements. Swift enums in Objective-C environments can significantly reduce boilerplate code while improving code readability and maintainability. For instance, when implementing state machines or managing application workflows, Swift enums provide a structured approach that minimizes the risk of runtime errors. The strong typing system ensures that only valid states can be represented, while the compiler's ability to enforce exhaustive switch statements helps prevent logical errors. These characteristics make "swift enum objc" integration an increasingly attractive option for developers seeking to modernize their Objective-C codebases while maintaining compatibility with existing systems.
How Does Swift Enum in Objective-C Work?
To fully grasp how Swift enums function within Objective-C environments, it's essential to understand the technical mechanisms that enable this cross-language interoperability. At the heart of this integration lies the @objc attribute, which serves as the bridge between Swift's modern enum implementation and Objective-C's traditional architecture. When a Swift enum is marked with @objc, the compiler automatically generates an equivalent Objective-C representation using NS_ENUM or NS_OPTIONS structures. This transformation process ensures that the enum's cases are mapped to integer values while preserving their semantic meaning across language boundaries. However, this interoperability comes with certain limitations – Swift enums with associated values cannot be directly exposed to Objective-C, requiring developers to implement alternative solutions for complex data structures. The code structure of Swift enums in Objective-C contexts follows a systematic approach that balances modern Swift features with Objective-C compatibility requirements. A typical implementation begins with defining the enum cases, often accompanied by raw values that facilitate seamless conversion between languages. For example, a simple Status enum might include cases like .success, .failure, and .pending, each assigned specific integer values. Developers can enhance these enums with computed properties and methods, although these additional features won't be directly accessible in Objective-C code. The bridging process creates an Objective-C interface that maintains the enum's basic functionality while omitting Swift-specific capabilities like pattern matching or associated values. Several technical challenges emerge when working with Swift enums in Objective-C environments. One primary concern involves memory management and reference counting, particularly when enums are used in performance-critical sections of code. The bridging process introduces additional overhead, as Swift enums must be converted to their Objective-C counterparts and vice versa. This conversion can impact performance, especially in tight loops or frequently called methods. Additionally, developers must contend with limited error handling capabilities, as Swift's powerful error handling mechanisms using enums cannot be directly translated to Objective-C. To address these challenges, experienced developers often implement wrapper classes or protocols that provide Objective-C compatible interfaces while maintaining Swift's advanced functionality behind the scenes. Despite these challenges, the integration of Swift enums into Objective-C projects offers significant advantages when implemented correctly. Developers can leverage Swift's type safety and compile-time checks while maintaining compatibility with existing Objective-C codebases. This approach enables gradual modernization of legacy systems, allowing teams to introduce Swift's powerful features without requiring complete codebase overhauls. The key to successful integration lies in understanding the limitations of the bridging process and designing enums with these constraints in mind. By carefully structuring enums and their associated functionality, developers can create robust, maintainable code that bridges the gap between Swift's modern capabilities and Objective-C's established ecosystem.
Read also:Shannon Doherty A Comprehensive Look At Her Life Career And Legacy
What Are the Benefits of Using Swift Enum in Objective-C?
The integration of Swift enums into Objective-C projects brings numerous advantages that significantly enhance both development efficiency and application quality. One of the most notable benefits is the substantial improvement in code readability and maintainability. Swift enums provide a clear, structured way to represent states and conditions, making the code more intuitive to understand and modify. This clarity becomes particularly valuable in large-scale projects where multiple developers work on the same codebase. For instance, when managing network request states, a well-defined Swift enum can encapsulate all possible states (like .loading, .success(data), .failure(error)) in a single, easily comprehensible structure. This approach reduces cognitive load for developers and minimizes the risk of introducing bugs during code maintenance. From a performance perspective, Swift enums offer several advantages that can lead to more efficient applications. The compiler's ability to optimize enum usage through techniques like memory layout optimization and static dispatch results in faster execution times compared to traditional Objective-C approaches. Additionally, the strong type system ensures that only valid states can be represented, eliminating the need for extensive runtime checks and reducing the potential for invalid state errors. These performance benefits become especially significant in resource-constrained environments like mobile devices, where efficient memory usage and quick execution are crucial for maintaining smooth user experiences. The impact on software architecture is perhaps the most transformative benefit of using Swift enums in Objective-C projects. By enabling developers to create more modular and reusable code components, Swift enums facilitate better separation of concerns and improved code organization. This architectural advantage manifests in several ways: first, enums can serve as powerful tools for implementing state machines, making complex workflows easier to manage and debug. Second, they provide a natural way to implement protocol-oriented programming patterns, allowing developers to create more flexible and extensible systems. Third, the ability to define methods and computed properties within enums enables better encapsulation of related functionality, leading to cleaner and more maintainable code structures. These architectural improvements not only enhance current development practices but also lay a solid foundation for future code evolution and feature expansion.
What Are the Common Challenges with Swift Enum in Objective-C?
Despite the numerous advantages of integrating Swift enums into Objective-C projects, developers often encounter several significant challenges that can impact project success. One of the most prevalent issues revolves around compatibility limitations, particularly when dealing with advanced Swift enum features. While basic enums can be bridged to Objective-C using the @objc attribute, more sophisticated capabilities like associated values and recursive enums cannot be directly translated. This limitation forces developers to implement workarounds, such as creating wrapper classes or using alternative data structures, which can introduce additional complexity and potential performance overhead to the project. Performance considerations present another crucial challenge in "swift enum objc" integration. The bridging process between Swift and Objective-C introduces additional computational costs, especially in scenarios requiring frequent conversions between the two representations. This performance impact becomes particularly noticeable in high-frequency operations or real-time systems, where the overhead of enum conversion can lead to noticeable latency. Developers must carefully evaluate these performance implications and potentially optimize critical code paths by minimizing cross-language conversions or implementing caching mechanisms to reduce the conversion frequency. Error handling represents a third significant challenge when working with Swift enums in Objective-C environments. Swift's powerful error handling mechanisms, which leverage enums to represent various error states, cannot be directly utilized in Objective-C code. This limitation requires developers to implement alternative error handling strategies that maintain compatibility with Objective-C's traditional approaches while still leveraging Swift's type safety benefits. Additionally, debugging issues related to enum conversions can be particularly challenging, as errors may manifest differently depending on whether they occur in Swift or Objective-C code. These challenges necessitate careful planning and thorough testing to ensure robust error handling across language boundaries. To address these challenges, developers must adopt strategic approaches that balance the benefits of Swift enums with the practical constraints of Objective-C environments. This often involves creating hybrid solutions that leverage Swift's advanced features where possible while maintaining compatibility with Objective-C's limitations. For instance, developers might implement a two-layer architecture where Swift enums handle internal logic and state management, while Objective-C compatible interfaces provide external access. Such approaches require careful design and thorough documentation to ensure maintainability and prevent confusion among team members working across both language environments.
Best Practices for Implementing Swift Enum in Objective-C
Successful integration of Swift enums into Objective-C projects requires adherence to several key best practices that can significantly enhance both development efficiency and code quality. One fundamental strategy involves establishing clear naming conventions that bridge both languages effectively. Developers should use descriptive, consistent naming patterns that align with both Swift's camelCase convention and Objective-C's traditional naming standards. For instance, prefixing enum names with specific identifiers (like "SE" for Swift Enum) can help distinguish them in mixed-language projects while maintaining readability across different codebases. This approach not only improves code organization but also facilitates easier debugging and maintenance. Optimizing code structure plays a crucial role in effective "swift enum objc" implementation. Developers should consider creating dedicated bridging modules that handle the conversion between Swift enums and their Objective-C counterparts. These modules can include utility functions for safe conversion, error handling mechanisms, and caching strategies to minimize performance overhead. Additionally, organizing enums into logical groupings based on their functionality or usage patterns can help maintain a clean project structure. For example, separating network-related enums from UI state enums not only improves code organization but also makes it easier to manage dependencies and update specific components without affecting others. Testing and debugging strategies require special attention when working with Swift enums in Objective-C environments. Implementing comprehensive unit tests that cover both Swift and Objective-C usage scenarios is essential for catching potential issues early in the development process. Developers should create test cases that verify enum conversion accuracy, handle edge cases, and test performance under various conditions. When debugging, it's crucial to understand how enums are represented in both languages and use appropriate tools to inspect their states. Xcode
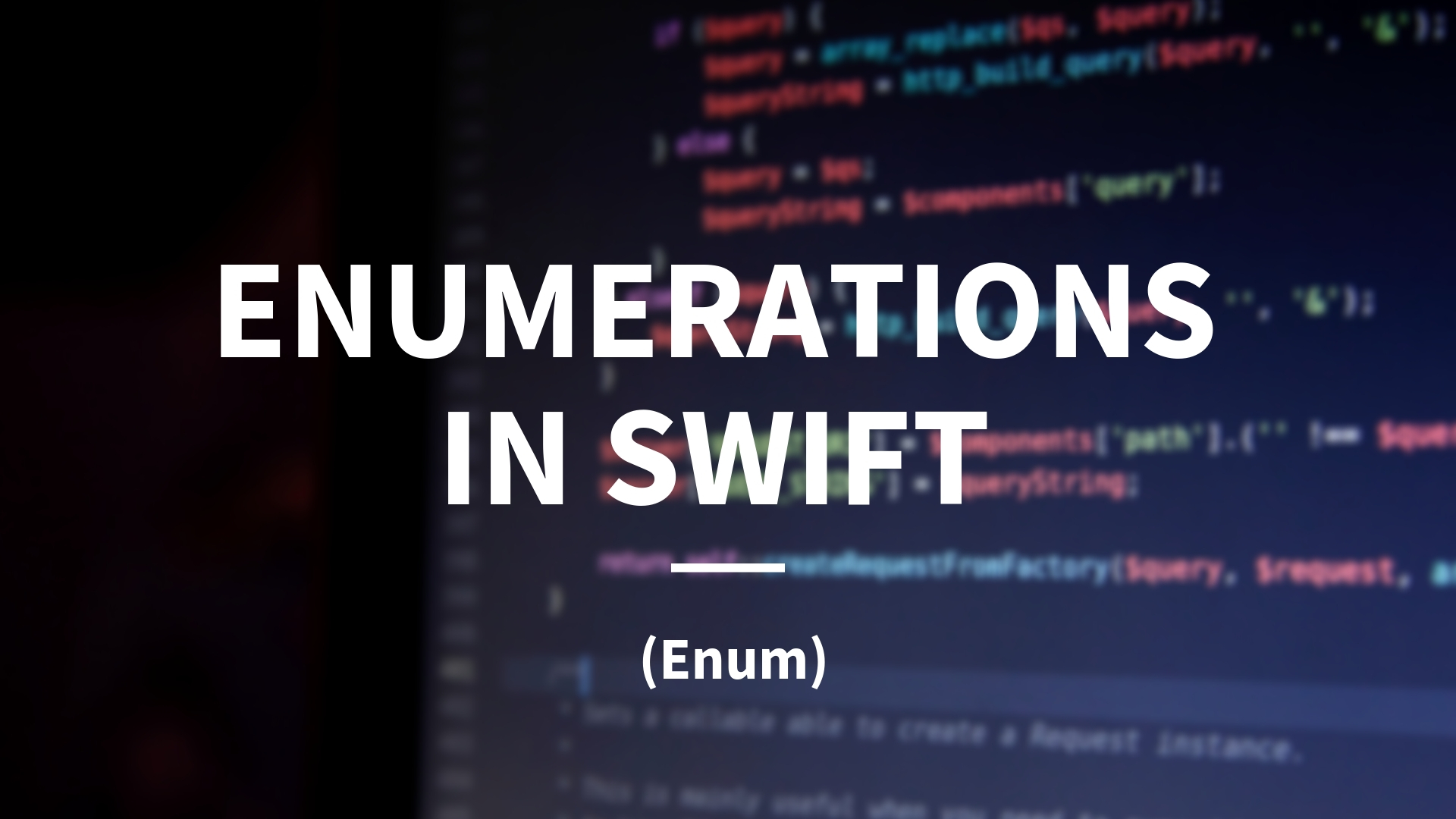
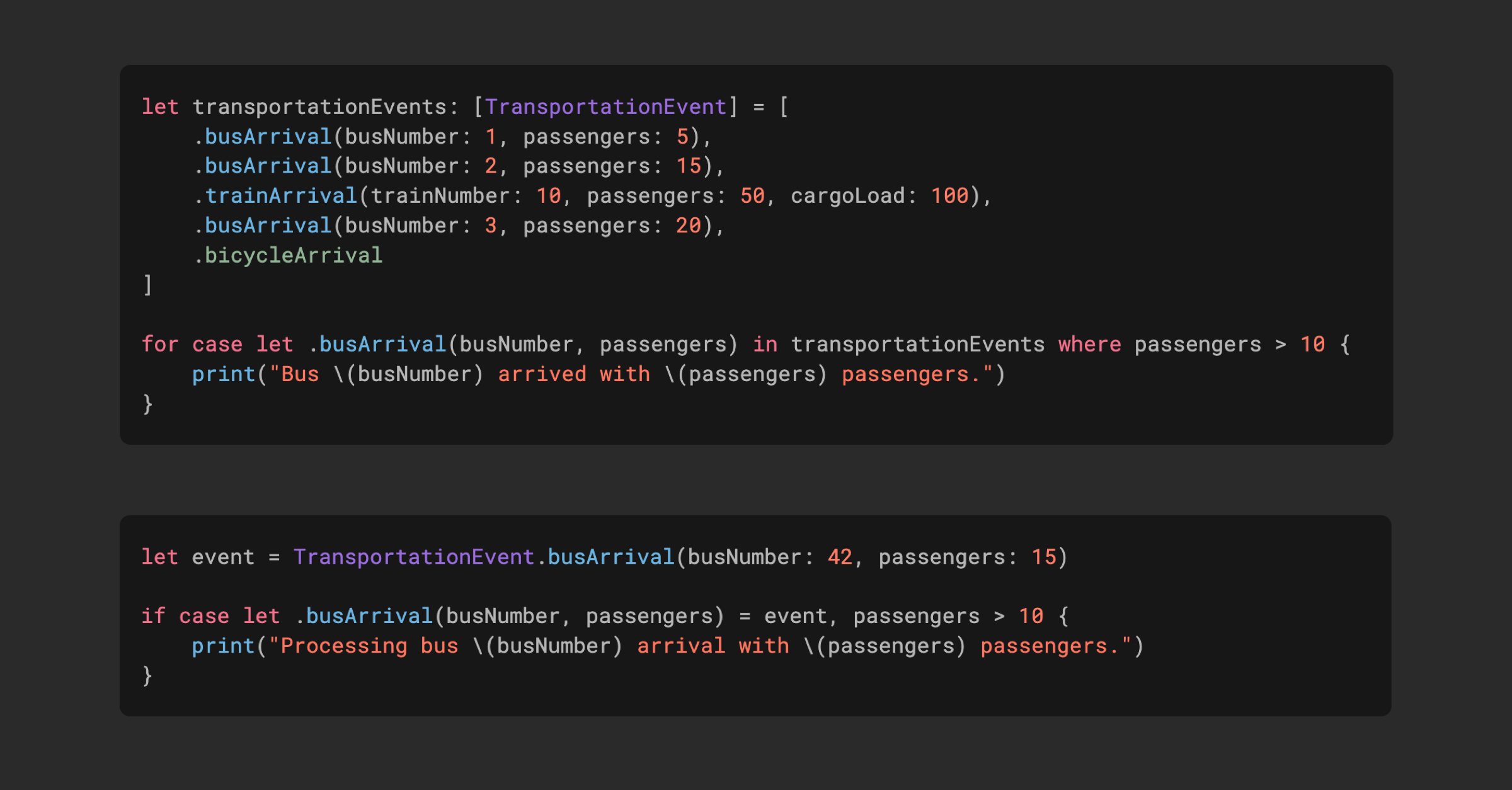