Objective-C enum, often referred to as enumerations, is a powerful feature that simplifies code readability and organization. Whether you're a beginner developer or a seasoned programmer, understanding how to effectively use enums can significantly enhance your coding efficiency. Enums provide a way to define a set of named values, making your code more intuitive and easier to maintain. With Objective-C being a cornerstone language for macOS and iOS development, mastering enums is essential for building robust applications. In this article, we will explore everything you need to know about Objective-C enum, from its basic syntax to advanced use cases, ensuring you walk away with actionable insights.
At its core, an Objective-C enum is a user-defined type that consists of a set of named constants. These constants are often used to represent a collection of related values, such as days of the week, states of a system, or error codes. By assigning meaningful names to these values, enums eliminate the need for magic numbers or strings in your code, reducing errors and improving clarity. As we delve deeper into this topic, you'll discover how enums can streamline your development process and make your codebase more maintainable. Whether you're building a small utility app or a large-scale enterprise solution, Objective-C enum is a tool you’ll want in your programming arsenal.
In today’s fast-paced development environment, writing clean, efficient, and maintainable code is more important than ever. Objective-C enum plays a crucial role in achieving this goal by providing a structured way to handle sets of related values. As we progress through this article, you’ll learn not only how to define and use enums but also how to leverage their full potential in various programming scenarios. From basic syntax to advanced techniques, this guide will equip you with the knowledge and skills needed to harness the power of Objective-C enum effectively.
Read also:Ullu Web Series Online Play Everything You Need To Know
Table of Contents
- What is Objective-C Enum and Why Should You Use It?
- How to Define an Enum in Objective-C?
- What Are the Practical Use Cases of Objective-C Enum?
- Enum vs. Macros: Which One Should You Choose?
- How to Use Advanced Techniques with Objective-C Enum?
- What Are the Common Mistakes When Using Objective-C Enum?
- Frequently Asked Questions About Objective-C Enum
- Conclusion: Why Objective-C Enum Matters
What is Objective-C Enum and Why Should You Use It?
Objective-C enum is a data type that allows developers to define a set of named constants. This feature is particularly useful when you need to represent a group of related values, such as the days of the week, states of a machine, or error codes. By using enums, you can replace hard-coded values with meaningful names, making your code easier to read and maintain. For example, instead of using the integer 0 to represent "Monday" and 1 for "Tuesday," you can define an enum called Weekdays
with named constants like Monday
and Tuesday
.
One of the primary benefits of using Objective-C enum is improved code readability. When you define an enum, you're essentially creating a contract within your codebase that specifies the possible values a variable can take. This reduces the likelihood of errors caused by using incorrect or undefined values. Additionally, enums can enhance code maintainability. If you need to add or modify values in the future, you can do so in one place, ensuring consistency across your application. This is particularly important in large projects where multiple developers are working on the same codebase.
Why Should You Use Objective-C Enum?
Using enums in Objective-C offers several advantages:
- Clarity: Enums make your code more readable by replacing cryptic numbers or strings with descriptive names.
- Type Safety: Enums help prevent invalid assignments by restricting variables to a predefined set of values.
- Maintainability: Centralizing related values in an enum makes it easier to update and manage your code.
- Performance: Enums are lightweight and efficient, as they are typically implemented as integers under the hood.
By leveraging these benefits, you can write code that is not only easier to understand but also less prone to bugs. Whether you're working on a small project or a large-scale application, Objective-C enum is a tool that can help you achieve cleaner and more efficient code.
How to Define an Enum in Objective-C?
Defining an enum in Objective-C is straightforward and follows a simple syntax. To create an enum, you use the typedef enum
keyword followed by the name of the enum and its constants. For example:
typedef enum { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday } Weekdays;
In this example, we've defined an enum called Weekdays
with seven constants representing the days of the week. By default, the first constant (Monday
) is assigned the value 0, and each subsequent constant is incremented by 1. However, you can explicitly assign values to the constants if needed:
Read also:Pink Heart Movie A Deep Dive Into Love Emotion And Cinematic Brilliance
typedef enum { Red = 1, Green = 2, Blue = 3 } Colors;
How to Use Enums in Your Code?
Once you've defined an enum, you can use it just like any other data type in Objective-C. For example, you can declare a variable of the enum type and assign it one of the predefined constants:
Weekdays today = Monday;
This approach ensures that the variable today
can only take one of the values defined in the Weekdays
enum. If you attempt to assign an invalid value, the compiler will generate an error, helping you catch mistakes early in the development process.
Can You Use Enums with Switch Statements?
Yes, enums work exceptionally well with switch
statements. Since enums represent a finite set of values, they are ideal for use in switch
cases. For example:
switch (today) { case Monday: NSLog(@"It's Monday!"); break; case Tuesday: NSLog(@"It's Tuesday!"); break; default: NSLog(@"Invalid day!"); }
This combination of enums and switch
statements makes your code more structured and easier to follow.
What Are the Practical Use Cases of Objective-C Enum?
Objective-C enum is not just a theoretical concept; it has numerous practical applications in real-world development. One common use case is representing states or modes in an application. For example, if you're building a music player app, you might define an enum to represent the playback states:
typedef enum { Stopped, Playing, Paused } PlaybackState;
This enum can then be used to control the behavior of your app, ensuring that only valid states are assigned to the playback variable. Another practical use case is handling error codes. By defining an enum for error codes, you can make your error-handling logic more robust and easier to understand.
How Can Enums Improve Your Code?
Enums can improve your code in several ways:
- Error Reduction: By restricting variables to predefined values, enums reduce the risk of invalid assignments.
- Code Clarity: Enums make your code self-documenting, as the names of the constants convey their purpose.
- Scalability: Adding new values to an enum is straightforward, making it easy to extend your codebase.
Enum vs. Macros: Which One Should You Choose?
When it comes to defining constants in Objective-C, developers often face a choice between enums and macros. While both options have their merits, enums are generally preferred for several reasons. First, enums are type-safe, meaning the compiler can catch errors related to invalid assignments. Macros, on the other hand, are simple text substitutions and do not provide the same level of safety.
Why Choose Enums Over Macros?
Here are some reasons why enums are a better choice than macros:
- Debugging: Enums are easier to debug because they appear in the debugger with their names, whereas macros are replaced with their values.
- Namespace: Enums provide a namespace for their constants, reducing the risk of naming conflicts.
- Readability: Enums make the code more readable by grouping related constants together.
How to Use Advanced Techniques with Objective-C Enum?
Objective-C enum supports several advanced techniques that can enhance its functionality. For example, you can use enums with bitwise operations to represent flags or options. This is particularly useful when you need to combine multiple values into a single variable.
Can You Use Enums for Bitwise Operations?
Yes, you can define an enum with powers of two to use it for bitwise operations:
typedef enum { OptionNone = 0, OptionA = 1
This allows you to combine options using the bitwise OR operator:
Options settings = OptionA | OptionB;
What Are the Common Mistakes When Using Objective-C Enum?
While enums are a powerful tool, there are some common pitfalls to avoid. One mistake is not initializing enum variables, which can lead to undefined behavior. Another is using enums for unrelated values, which can make your code harder to understand.
How to Avoid These Mistakes?
To avoid these mistakes, always initialize your enum variables and ensure that the constants within an enum are logically related.
Frequently Asked Questions About Objective-C Enum
What is the Purpose of Objective-C Enum?
Objective-C enum is used to define a set of named constants, improving code readability and maintainability.
Can Enums Be Used with Switch Statements?
Yes, enums work well with switch
statements, making your code more structured and easier to follow.
Are Enums Type-Safe?
Yes, enums are type-safe, meaning the compiler can catch errors related to invalid assignments.
Conclusion: Why Objective-C Enum Matters
Objective-C enum is a powerful feature that can significantly enhance your code's readability, maintainability, and efficiency. By mastering enums, you can write cleaner, more robust code that is easier to understand and maintain. Whether you're a beginner or an experienced developer, enums are a tool you’ll want in your programming arsenal.
For more information on Objective-C, check out this official Apple documentation.
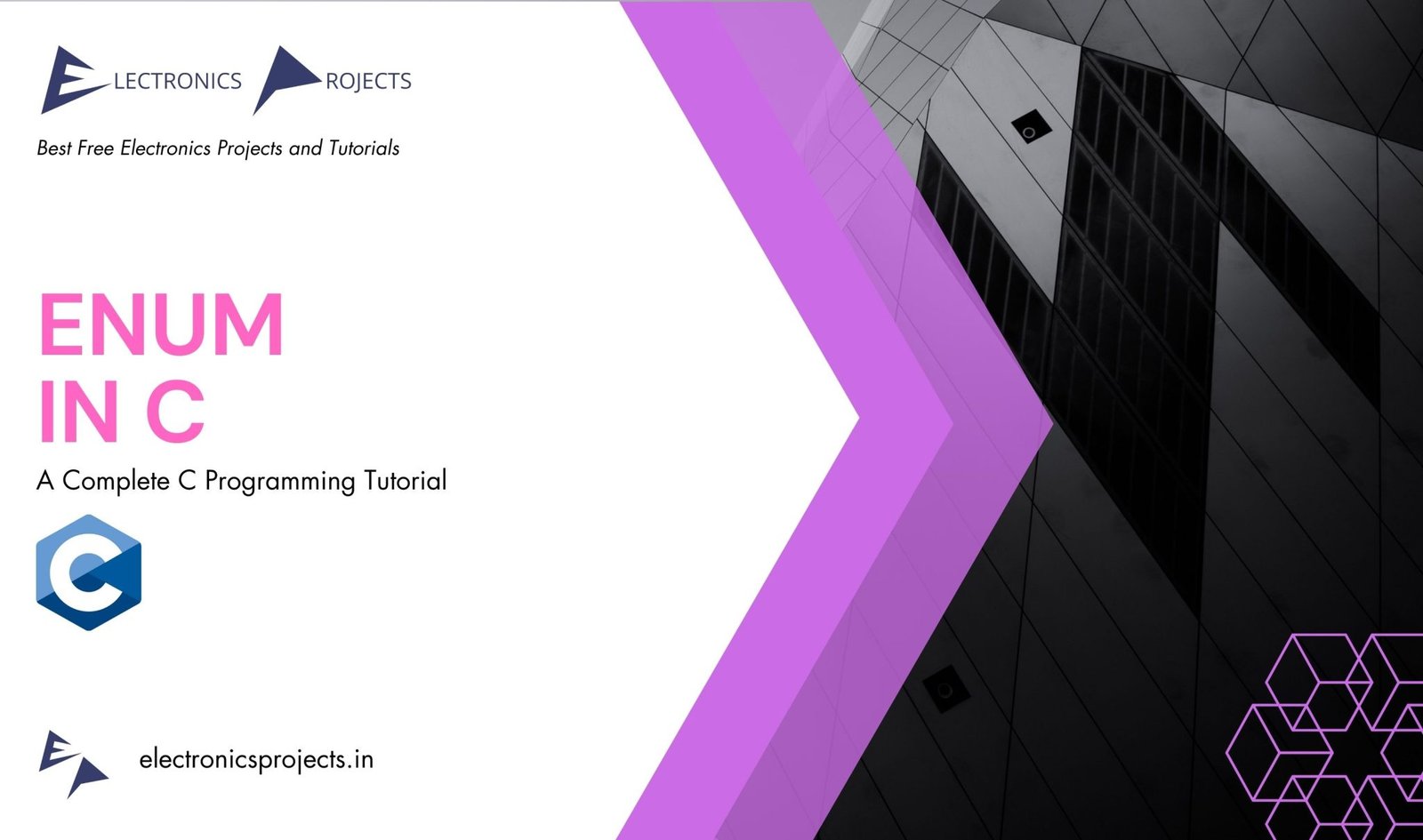
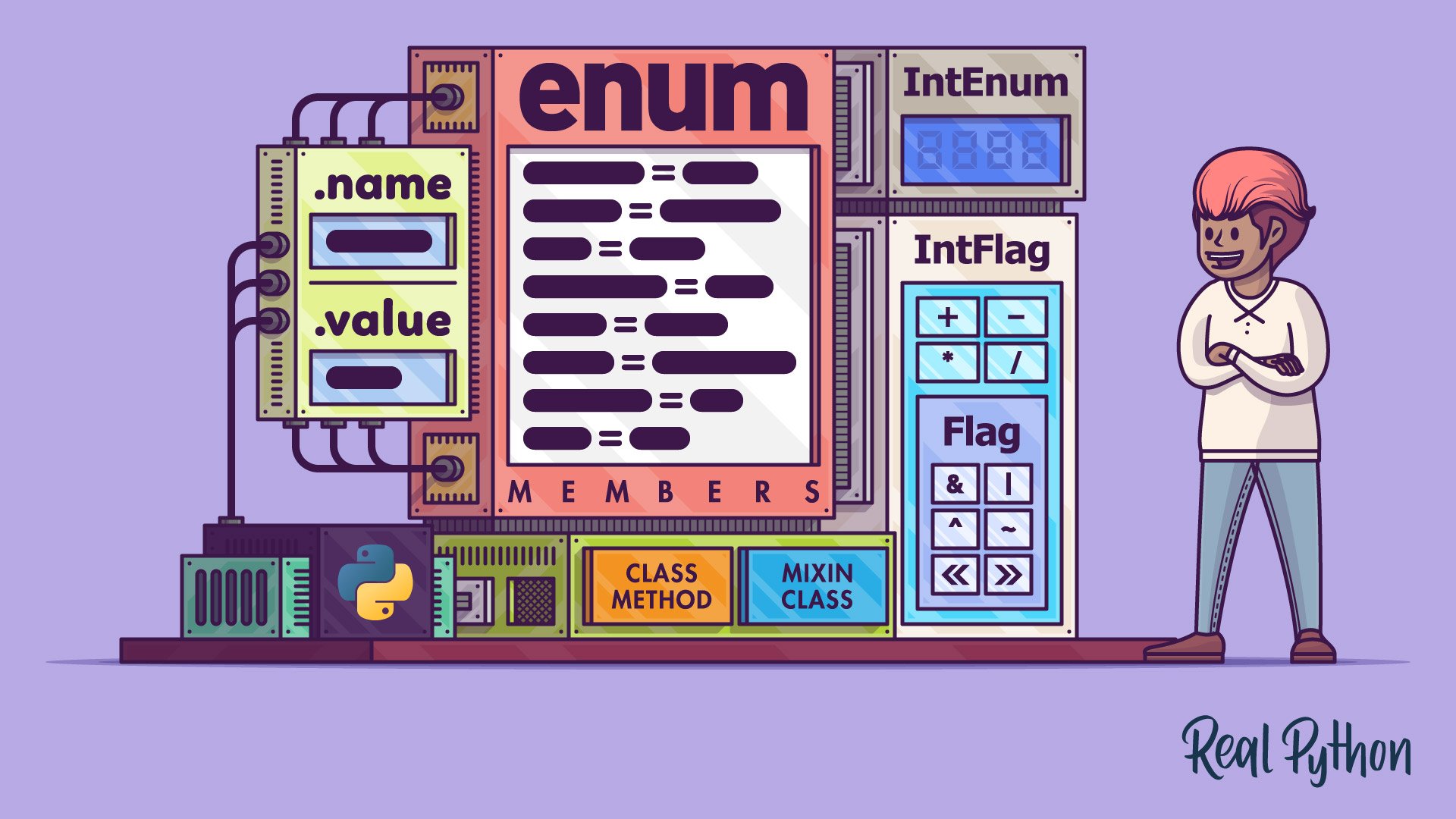